Agent Creator App API
The Agent Creator App allows seamless integration of AI-driven chat capabilities into your system using the Newo chat connector. This guide provides a step-by-step walkthrough on setting up, initializing, and handling real-time events.
Getting Started
Create a Newo Chat Connector
- Navigate to the Integrations page:
builder.newo.ai/integrations
. - Click the plus (+) button to create a new Newo Chat integration.
- Provide the required details:
idn
: Unique identifier for the connector.title
: Name of the integration.Domain
: The domain where the widget or app will be placed:- Use
http://localhost
for local development. - Use
https://example.com
for production.
- Use
- After saving, a
CLIENT_SECRET
will be generated and displayed in the modal. ThisCLIENT_SECRET
is required to make API requests to Newo.
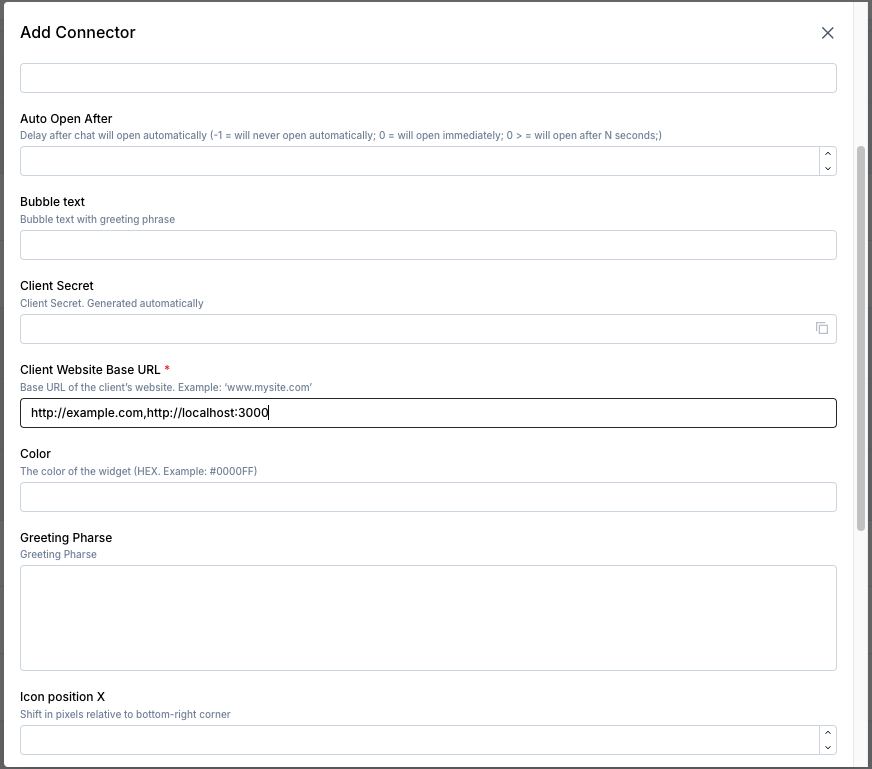
Initialization
To communicate with the connector, you need to obtain its connector_idn
and establish a WebSocket connection for real-time updates.
Retrieve Connector Settings
GET https://chat.newo.ai/api/internal/v1/talking-head/chat-settings?client_secret=CLIENT_SECRET
type ConnectorSettings = {
connector_idn: string
};
Create a Customer Actor
To identify customers in the Newo system, create an actor using the following request:
POST https://chat.newo.ai/api/internal/v1/talking-head/create-actor
type CreateActorPayload = {
name: string; // Customer actor's name
external_id: string; // Unique identifier of your customer (external_customer_id)
connector_idn: string; // Connector IDN
client_secret: string; // Client secret
};
Establish WebSocket Connection
To track the parsing progress and receive data updates, establish a WebSocket connection using socket.io:
io("https://chat.newo.ai/", {
transports: ['websocket'],
query: {
client_secret,
origin: globalThis.origin, // webpage origin e.g. https://example.com
external_id
}
});
Parsing Events
Start Parsing Data
POST https://chat.newo.ai/api/internal/v1/talking-head/send-actor-event
type ParsingRequestPayload = {
client_secret: string;
connector_idn: string;
event_idn: "onboarding_started";
external_id: string;
arguments: Array<{
name: string;
value: string;
}>;
};
Arguments:
referral
: Email address of the referral source.name
: Name of the customer.email
: Email address of the customer.phone_number
: Phone number of the customer.source
: Either a website URL.country_code
: The country code associated with the customer.external_customer_id
: Your customer ID.query_params
: Additional query parameters in JSON format.
WebSocket Events
As soon as parsing begins, socket events notify about the progress.
notify_scraping_step Event
Provides real-time updates during the parsing process.
type NotifyScrapingStepEvent = {
integration_idn: "newo_chat";
connector_idn: string;
command_idn: "notify_scraping_step";
external_event_id: string;
arguments: Array<{
name: string;
value: string;
}>;
};
Arguments:
user_actor_id
: Unique identifier of the customer actor.question
: The specific data point being parsed (e.g., business_working_hours).answer
: The extracted response to the question.step_number
: The current parsing step number.max_steps
: The total number of steps in the parsing process.stage
: The processing stage (preprocessing, stage, postprocessing).
notify_scraping_finished Event
Triggered when a new customer has been successfully onboarded.
type OnboardingFinishedEvent = {
integration_idn: string;
connector_idn: string;
command_idn: "notify_scraping_finished";
external_event_id: string;
arguments: Array<{
name: string;
value: string;
}>;
};
Arguments:
user_actor_id
: Unique identifier of the customer actor.business_name
: Name of the business extracted from the data.agent_name
: Name of the AI agent assigned to the customer.agent_title
: Role/title of the AI agent (e.g., AI Host).industry
: Industry category associated with the business.
Checking Processing Status
To check the processing status, send the following request:
POST https://chat.stg.newo.ai/api/internal/v1/talking-head/send-actor-event
type ProcessingStatusRequest = {
client_secret: string;
connector_idn: string;
external_id: string;
event_idn: "agent_request";
arguments: [];
};
agent_info Event
Triggered after requesting the processing status.
type AgentInfoEvent = {
integration_idn: string;
connector_idn: string;
command_idn: "agent_info";
external_event_id: string;
arguments: Array<{
name: string;
value: string;
}>;
};
Arguments:
user_actor_id
: Unique identifier of the customer actor.phone_number
: The phone number associated with the customer.created_customer_idn
: The unique identifier of the newly created customer.state
: Current processing state (start, created, etc.).email
: Email address of the customer.business_name
: Name of the business associated with the customer.agent_name
: Name of the AI agent assigned to the customer.agent_title
: Role/title of the AI agent (e.g., AI Sales Rep).
This section allows users to request and monitor the processing status of their onboarding process in real time.
Agent Creation Completion Event
onboarding_finished Event
Returns details about the newly created customer and assigned AI agent.
type OnboardingFinishedEvent = {
integration_idn: string;
connector_idn: string;
command_idn: "onboarding_finished";
external_event_id: string;
arguments: Array<{
name: string;
value: string;
}>;
};
Arguments:
user_actor_id:
: Unique identifier of the customer actor.phone_number:
: AI agent phone number.created_customer_idn
: The unique identifier of the newly created customer.email
: Email address of the customer.industry
: Industry category of the customer's business.business_name
: Name of the business associated with the customer.agent_name
: Name of the AI agent assigned to the customer.agent_title
: Role/title of the AI agent (e.g., AI Host).
onboarding_error Event
Triggered when an error occurs during the onboarding process.
type OnboardingErrorEvent = {
integration_idn: string;
connector_idn: string;
command_idn: "onboarding_error";
external_event_id: string;
arguments: Array<{
name: string;
value: string;
}>;
};
Arguments:
description
: A message describing the error that occurred.
This marks the completion of the customer registration process, making the AI agent ready to interact with the newly onboarded customer.
Updated 2 months ago